Accelerometer:
How To
Rohan
Varma, Steven Cen
This tutorial will walk you
through creating a program that displays the movement of the device on the x,
y, and z axes (each axis represents a different kind of movement).
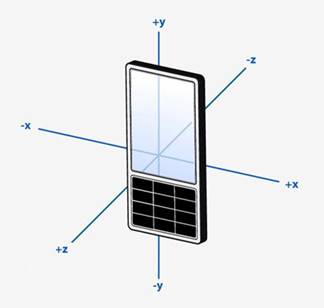
1. Set Up the Project.
- Create new project
- Since the accelerometer is being
used, the phone will be shaken in many ways, so it is a good idea to lock
the screen rotation in one direction. To do this we will alter the
manifest by putting the line android:screenOrientation=”portrait”
below
the line <activity android:name=”yourName” Your manifest
should now look like this
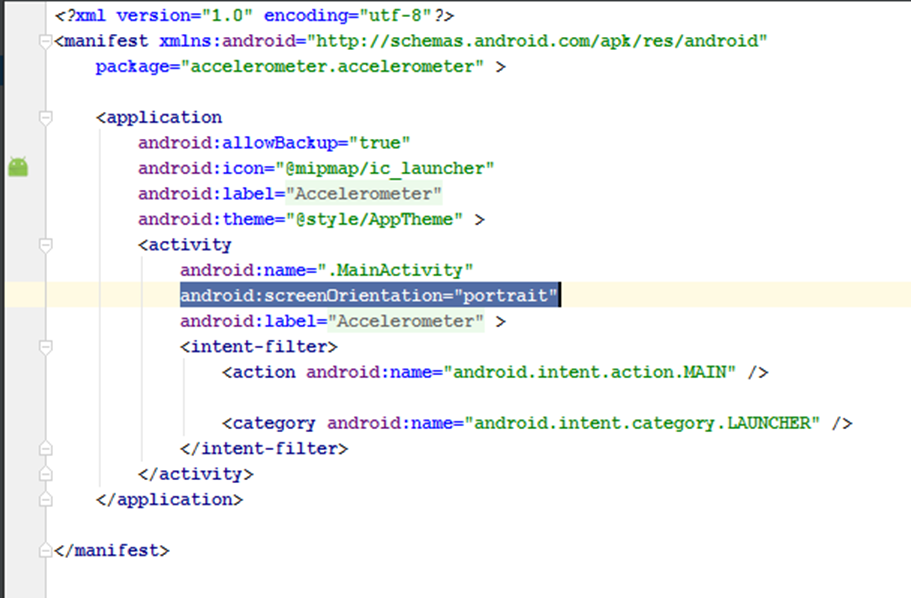
- In order to
display the x, y, and z values, create three textViews in your code. In my
code examples, they will show up as xView, yView, and zView.
2. Set Up the Sensor
- Make the main class implement the
SensorEventListener so that the accelerometer can be used

- There should be a red line underneath
that line, do an Alt+enter on it and it will ask you to implement methods.
Click on that and these two methods should appear on it
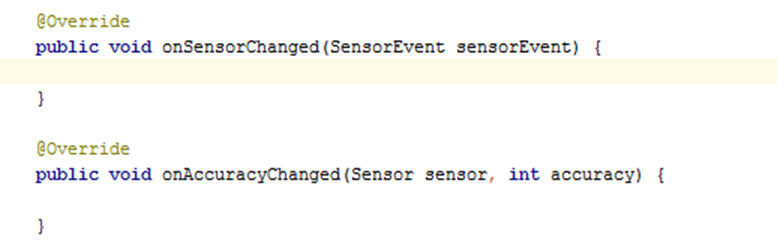
- In order to use the accelerometer, we
also need to create two objects: a SensorManager and a Sensor. Declare
these two objects just below the Main header

- Now we need to initialize these
objects in the onCreate method your onCreate method should look like this
(remember that xView, yView, and zView are the TextViews I created at the
beginning)
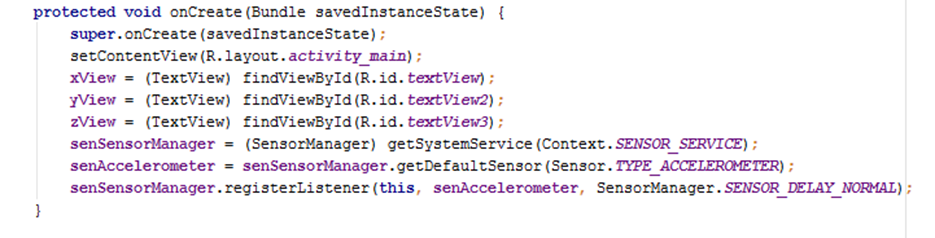
Those
last three lines initialize the variables that will track your phones movement
using the accelerometer.
- Now, in order to ensure that the
accelerometer works well even if the app is closed/paused and then
restarted/resumed, add these two methods. They unregister and re-register
(essentially remove and recreate) the SensorManager when the app is
closed/restarted
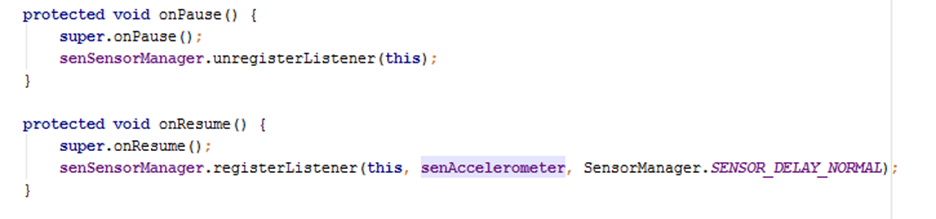
3. Detecting the Movement
- Now we will actually detect the
gestures of the phone. Most of the logic will be done in the
onSensorChange method but before that, there are a few variables to be
declared below the Main header

- In order to actually get the current
x, y, and z values, add the following code into your onSensorChanged
method
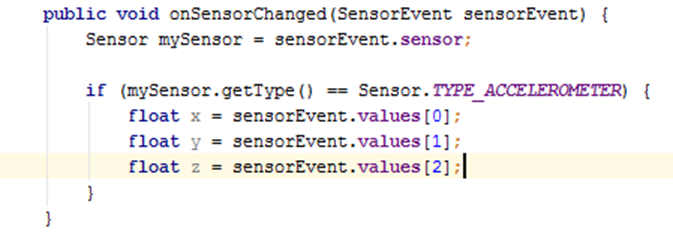
- Now we are getting the x, y, and z
values, but the device’s sensor is extremely sensitive and is getting
slightly different readings even if you think you are holding the device
still. As a result, the onSensorChanged method is being called multiple
times per second, but we don’t want all this data. So we now add code that
makes sure at least 100 milliseconds have passed since the last time
onSensorChanged method was run. We do this by storing the systems current
time in curTime and checking it against lastUpdate (which was declared
earlier)
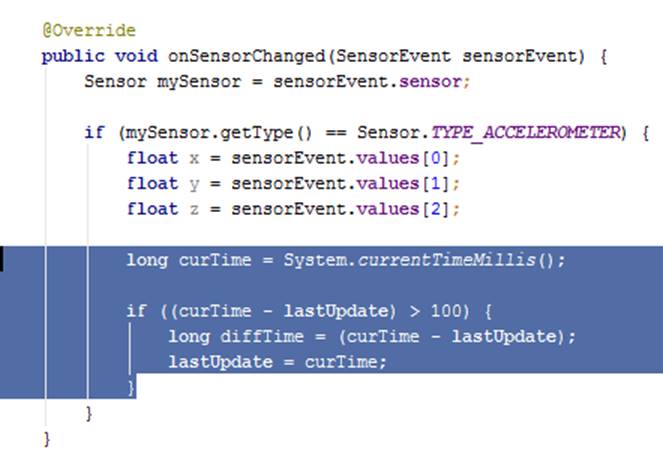
Your
onSensorChanged method should now look like this
- The last
thing we need to do now is actually detect when the device has been moved.
We do this by calculating the speed of the device by using Math.abs and
then comparing it to the SHAKE_THRESHOLD int we declared earlier. If the
speed is greater than the SHAKE_THRESHOLD the program will recognize that
the device has been moved and will display the x , y, and z values.
However, if the speed is smaller than the SHAKE_THRESHOLD, the sensor will
do nothing. Because of this, the value that you set the SHAKE_THRESHOLD to
determines how sensitive the sensor is, and by changing this value you
change the sensitivity of the sensor.
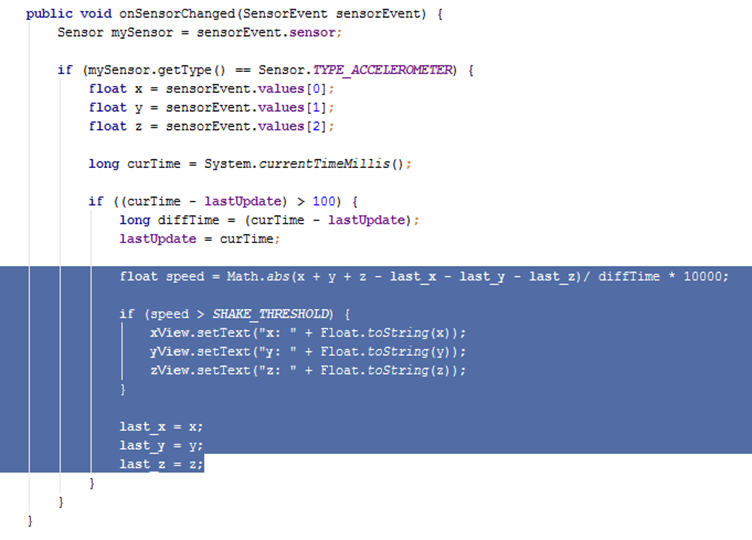